Lab 1: C, Python and Matlab
This lab is part of Assignment 1. You are to complete the exercises here before arriving in lab on Monday/Tuesday. In lab, you and your partner will demonstrate your code to your TA, and get feedback. Your revised code is due on January 24 at noon.
Your partner must be in the same lab group as yourself. Every student is assigned to a single lab group within their lab section. The lab groups are posted on Piazza.
As always, your code must comply with the CS 190 Style Specifications, and work on the ECF machines. The marking scheme for Assignment 1 is available here.
Part Zero: SVN Setup
Go to https://stanley.cdf.toronto.edu/markus/csc190-2013-01 and log in wih your ECF account. When you log in, it will list "Your Assignments"; click on "Assignment 1"
It will look like:
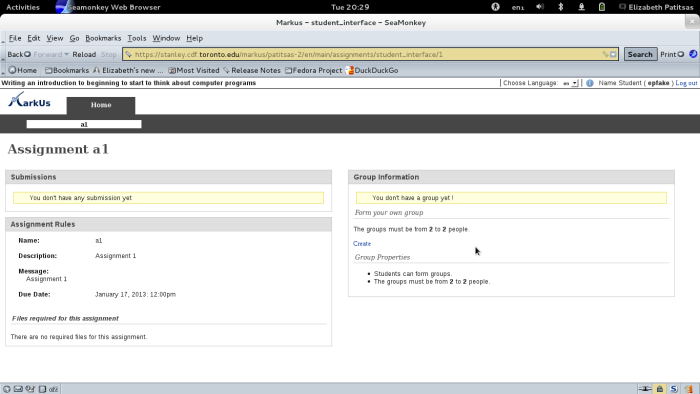
Under "Form your own group", click "Create". Then click the Invite button. There, enter the ecf username of your partner. (Important: they must not have hit "Create" in their instance of MarkUs, else you cannot invite them!)
Your partner should now log in to MarkUs and navigate to the Assignment 1 Group Information. It will now display the invitation:
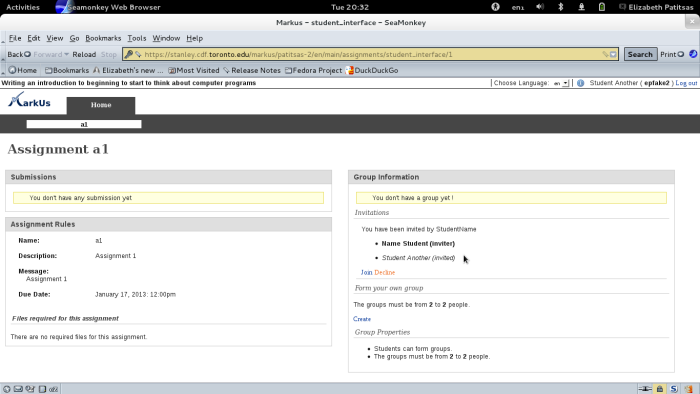
Click "Join" to finalize your group. Now that you have a group, Group Information will display your group's SVN repository. It will look like: https://stanley.cdf.toronto.edu/markus/csc190-2013-01/group_0000. Learn about SVN here.
Subversion, or SVN, is a version control system. This is software that logs the different changes made to a file, and allows for collaborative editing. To set up your repository, go to a terminal, navigate to where you want the repository created, then svn checkout https://stanley.cdf.toronto.edu/svn/csc190-2013-01/group_0000
It will ask you to log in using the username of the computer you are currently logged in to. If this is not the same as your ECF login, simply hit enter, and it will ask you to provide a username. Give it your ECF login.
Once you have checked out the repository, you can add files using the svn add FILENAME command. To commit to the SVN repository, use the command svn commit -m "commit message goes here". Learn about using SVN on the command-line here. (Note: it is permissible to use a graphical SVN client, like SmartSVN or TortoiseSVN; however, the CS 190 teaching staff will only support command-line svn.)
Important: when you check out your repository, it will contain a folder, "a1" -- your TAs will only be able to see (and mark) the contents of this folder. The contents of this folder will also be viewable when you log into the MarkUs website.
Tip: compile and test as you go; commit new versions regularly as you complete each TODO item.
Part One: Python to C
Download and read the file mean_temps.py; this contains Python code to calculate the average temperature of each season in Toronto.
TODO: Your task is to translate that program into working C code. Download the file mean_temps.c to begin.
Next, we want to compare the runtime of these two programs, using the time utility. Which is faster: C or Python? Record the measurements you get; you will use this for your report.
Commit your code at this point, if you have not already.
Part Two: Matlab to C
Download the Matlab scripts array_traversal_col.m and array_traversal_row.m. These files both create a 1773x1773 array of ones, and print the sum of all the elements. The difference between them is in how they iterate over the array: whether to iterate over columns then rows, or over rows then columns.
TODO: Your task is to write two C programs, array_traversal_col.c and array_traversal_row.c, which do the same thing in C.
Once you have done this, execution/runtime of all four programs, and record the results you get in a table like the one below. To time the Matlab script on the command-line, use the commands:
time matlab -nodisplay -nosplash -nojvm <
array_traversal_col.m
time matlab -nodisplay -nosplash -nojvm <
array_traversal_row.m
C code |
Matlab code |
|
Column-major traversal (in ms) |
||
Row-major traversal (in ms) |
Was there a difference between the C programs? If so, which was faster? What about the two Matlab programs? Is C faster than Matlab? Document this for your report.
Demonstrate your code to your TAs in lab, as well as your ability to commit code to your SVN repository.
When you are done, you should begin working on Lab 2. If you have no revisions to make to Lab 1, or complete them, after talking to your TA, you should spend your time in lab working on Lab 2.