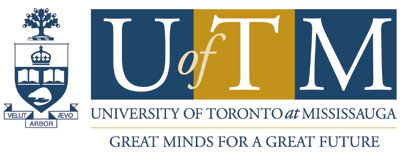 |
CSC148 Introduction to Computer Science
Assignment 1 Object Recursion, OO Programming
|
Due: |
Sunday Feb 24, 11:50PM
|
Late penalty: | 20% penalty for 48 hours late, not excepted after that.
|
Hand in: |
Electronic submit here.
|
Groups: | Work in groups of size one. |
Environment: | We will test your code under python in the lab. |
Warehouse Wars
You will be writing a larger application using the Object Oriented paradigm, Python and PyGame (installed in our lab).
The game you will be building, Warehouse Wars, was first built by a group of us while in highschool.
I believe David Foster,
created it first, and then we each re-wrote it and added our own features.
This top down game takes place in a warehouse.
The player, is driving a forklift and can push boxes around the warehouse.
The player can in fact push rows of boxes, vertically, horizontally or diagonally.
Unfortunately there are monsters in the warehouse roaming around. If they touch the player,
the player looses a life. These monsters have a weakness. They must be able to move.
If they are surrounded, for a certain amount of time, they loose their life and disappear.
The forklift drivers jobs is to move boxes around the warehouse
to surround each monster on all sides and remove them.
Just a reminder, the forklift can push more than a single box at a time, it can push
a whole row, column or diagonal collection of boxes at a time, being forced to
stop only when the leading box reaches an obstruction (ie. monster or wall or the end of the stage).
For CSC148, Warehouse Wars consists of a few parts: A lab, and an assignment.
The Assignment (done individually)
Note: When you are finished writing all of your code, draw and submit the UML Diagram for all of the
Warehouse Wars classes. You can do this with Dia in the lab, or on paper and scan and submit
it to us electronically.
The starter code can be found here.
Before drawing the UML diagram, you are to
add some additional features, some required and others optional.
The ones with an (R) are required. All others are suggestions. Please
submit a readme.txt file including a list of all features
you implement.
Monsters:
Enhancements:
(R) Modify the existing Monster so that they
die when surrounded by boxes on all sides.
(R) Modify the existing Monster so that, when they hit another
actor, they bounce back (return in the same direction
they came).
Types:
(R) At least three different types of monsters. Two
in addition to the standard type above.
Below are some suggestions.
Monsters that can be pushed
Monsters that can be squished and then die. They can be pushed by
boxes until they can't be pushed further, and then the die.
Monsters that die when the player touches them
Monsters that randomly change direction
Monsters that find a direction that they can move
Monsters that, when they die, explode, removing boxes
Monsters that, when they die, explode, removing boxes and evolve into smarter monsters
Monsters that try to follow the player, wherever they are
Monsters that try to follow the player, when they 'see' them
Monsters that explode after living for some time
Monsters that explode when they get close enough to the player
Monsters that camoflage themselves as boxes for some time
Monsters that run away from the player
Boxes/Walls:
Types:
(R) Boxes that can be moved/pushed.
(R) A Wall, that is, an Actor that can't be moved
(R) Boxes that are sticky, so that when a monster moves into it or it gets
moved into a monster, the monster ends up being stuck to the box
and can't move.
KeyboardPlayer:
Features:
(R) KeyboardPlayer dies if monster 'touches' them. The Monster has to ask the
KeyboardPlayer to move. Similarly, if a KeyboardPlayer
asks a monster to move, the KeyboardPlayer dies. This makes
it possible for a Monster to walk right past a KeyboardPlayer.
It also makes your code simpler.
The exception to this is obviously if you create a type of Monster
that specifically dies when the player touches it.
(R) Add diagonal movement to the player
Change player icon depending on the direction they are moving
Two player game, both players try to kill the monsters
Add keyboard repeat behavour, so the player need only hold a key
Switch to numeric keypad, if available
Player can transport a limited number of times.
Misc:
(R) Game over
(R) Nothing can move off the stage
(R) Different icons for different types of things
Different icons for different direction movement
Animations for actors.
Marking
You should document each class
in the assignment, describing its role. Document any interesting methods, describing
what they do, not how they do it. Document any interesting algorithms. Name variables,
methods etc. so that minimal documentation is necessary. Poor documentation can
reduce your marks in the following marking scheme.
The marking will be as follows, required features will get a mark out of 5,3, or 1
depending on the complexity of the feature.
Complex feature:
5/5 Simple, clear, concise code, making effective use of the OO paradigm.
4/5 Works, but not simple code, does not make effective use of the OO paradigm.
3/5 Some significant issue or no use of the OO paradigm. Feature does not work.
2/5
1/5 Some attempt at implementing the feature.
Simple features:
3/3 Simple clear, concise solution
2/3 Not simple, or works in most cases
1/3 Some real attempt to implement the feature
Very Simple feature
1/1 it works
0/1 it does not work
Additional features and appearance will be given a total mark out of 5.
Implementing many extra features does not necessarily
gain you more marks. You shold balance the features with
the usability and fun involved in the game. A better looking,
interesting, fun game gets best marks.
References
Questions and Answers
- Question:
-
How can I work on this over the break?
- Answer:
-
- You can go into the lab. It will be open over the break.
- You can 'ssh' into the lab, or into cslinux. For example, from a ubuntu machine, bring up a command prompt and
ssh -Y UTORID@cs32.utm.utoronto.ca
you will then have a command prompt on lab machine 32 (for example). If you are running windows, you might want to install cygwin and then install X. Older Macs come with X installed. Newer ones
point you to an installation of X.
- You can
- install virtualbox (free) or vmware server (I have to get the license from Sue for you)
- Then install Ubuntu
- Install python3 on your Ubuntu virtual machine.
sudo apt-get install python3
- Finally, install pygame as outlined in here on your virtual machine.
- Go to the Ubuntu software center and install the development environment of your choice. Make sure it is python3 compatible.
- Question:
-
Will there be office hours over the break?
- Answer:
-
Yes, when is a good time for you?
- Question:
-
Can you clarify the sticky boxes.
- Answer:
-
When a monster touches a sticky box, or a sticky box is pushed into a monster,
it gets stuck to the box. This means that the monster can't move away from the box.
Sticky boxes can not push monsters. If a sticky box is pushed away from a monster,
the monster is free to move once again.
- Question:
-
Can you clarify when a moster dies.
- Answer:
-
At a high level, a monster is alive if it could potentially move to one of the surrounding spaces this includes a space occupied
by the player.
A monster is dead if all of the adjacent squares are unavailable, either out of bounds, or occupied by a non-keyboard player actor. It
is alive if at least one adjacent square is available.
- Question:
-
Any general design principles (Arnold asks himself)?
- Answer:
-
- Don't repeat yourself. If you find yourself doing the same thing in many places, make a method/function or use inheritance.
- 0,1,2=N: In programming, typically, Not doing something is different from doing something for one, but doing something
for two or three is probably not much different from doing something for the general case.
Example:
def min0():
return None
def min1(a):
return a
def min2(a,b):
if ab:
m=b
if m>c:
m=c
return m
def min4(a,b,c,d):
m=a
if m>b:
m=b
if m>c:
m=c
if m>d:
m=d
return m
# I give up...might as well find the min for an arbitrary list
def minL(L):
if L==[]:
return None
m=L[0]:
for e in L:
if m>e:
m=e
return m
- Code so that it is simple, clear and obvious. It is not easy to get to this point, but you will know when you get there.
All of a sudden, the code will not be confusing.
- Question:
-
I am having this funny problem when going through a list...
- Answer:
-
The following two do very different things. In fact the first
does not even do what you might expect it to do. See the 7 is still in the list.
The problem: Modifying a list while iterating over it.
def remove1(L):
for e in L:
print(e, 2*e+1)
if e%2==0:
L.remove(e)
if 2*e+1 in L:
L.remove(2*e+1)
print(L)
def remove2(L):
L2=L[:]
for e in L2:
print(e, 2*e+1)
if e%2==0:
L.remove(e)
if 2*e+1 in L:
L.remove(2*e+1)
print(L)
L=list(range(10))
remove1(L)
L=list(range(10))
remove2(L)
OUTPUT BELOW -----------------------------------------------------------------------
(0, 1)
(3, 7)
(4, 9)
(6, 13)
(8, 17)
[2, 3, 5, 7]
(0, 1)
(1, 3)
(2, 5)
(3, 7)
(4, 9)
(5, 11)
(6, 13)
(7, 15)
(8, 17)
(9, 19)
[3, 7]
- Question:
-
Does isinstance 'really' work?
- Answer:
-
Yes, consider...
class A:
def __str__(self):
return('instance of A')
class B(A):
def __str__(self):
return('instance of B')
class C(B):
def __str__(self):
return('instance of C')
class D(C):
def __str__(self):
return('instance of D')
a=A()
b=B()
c=C()
d=D()
for z in [a,b,c,d]:
for Z in [A,B,C,D]:
print(str(z), Z.__name__, isinstance(z,Z))
OUTPUT BELOW -----------------------------------------------------------------------
('instance of A', 'A', True)
('instance of A', 'B', False)
('instance of A', 'C', False)
('instance of A', 'D', False)
('instance of B', 'A', True)
('instance of B', 'B', True)
('instance of B', 'C', False)
('instance of B', 'D', False)
('instance of C', 'A', True)
('instance of C', 'B', True)
('instance of C', 'C', True)
('instance of C', 'D', False)
('instance of D', 'A', True)
('instance of D', 'B', True)
('instance of D', 'C', True)
('instance of D', 'D', True)
- Question:
-
Any advice on installing pygame?
- Answer:
-
Filip pointed me to this and says to search for
pygame
.